How to Use PrismJS with NextJS
This is a summary of how I got syntax highlighting to work on my NextJS blog
Install Prism
First I installed Prism.JS from NPM. You can use Yarn
npm i prismjs
or
yarn add prismjs
Import Prism into the Next.JS Component
Next, I imported Prism and the React useEffect hook into the blog details page.
The Okaidia theme looks good so, I imported its CSS styles.
Then, I called the Prism.highlightAll function inside the useEffect hook. This will turn on syntax highlighting after the component is mounted
Finally, I added a className specifying the programming language e.g. className="language-css" or className="language-js"
import React, { useEffect } from "react"; import Prism from "prismjs"; import "prismjs/themes/prism-okaidia.css"; import styles from "styles/Details.module.scss" const SingleProject = ({ project }) => { useEffect(() => { Prism.highlightAll() }, []) if (!project) return return ( <> <div className={styles.Container}> <div dangerouslySetInnerHTML={{ __html: project.description }} className={`${styles.formatter} language-js`} > </div> </div> </> ) } export default SingleProject
Make it your way
Prism provides several customization options. You can download a custom minified CSS from https://prismjs.com/download.html and import it inside your _app.js
// SingleProject.js import React, { useEffect } from "react"; import Prism from "prismjs"; import styles from "styles/Details.module.scss" const SingleProject = ({ project }) => { useEffect(() => { Prism.highlightAll() }, []) if (!project) return return ( <> <div className={styles.Container}> <div dangerouslySetInnerHTML={{ __html: project.description }} className={`${styles.formatter} language-js`} > </div> </div> </> ) } export default SingleProject
My _app.js file looks like this
// _app.js import Head from "next/head" import Layout from "../components/Layout" import "../styles/globals.scss" import "../styles/prism.css" function MyApp({ Component, pageProps }) { return ( <Layout> <Head> <link rel="shortcut icon" href="/assets/favicon.ico" /> </Head> <Component {...pageProps} /> </Layout> ) } export default MyApp
We're done! You may need to refresh your page to activate Prism.
The result
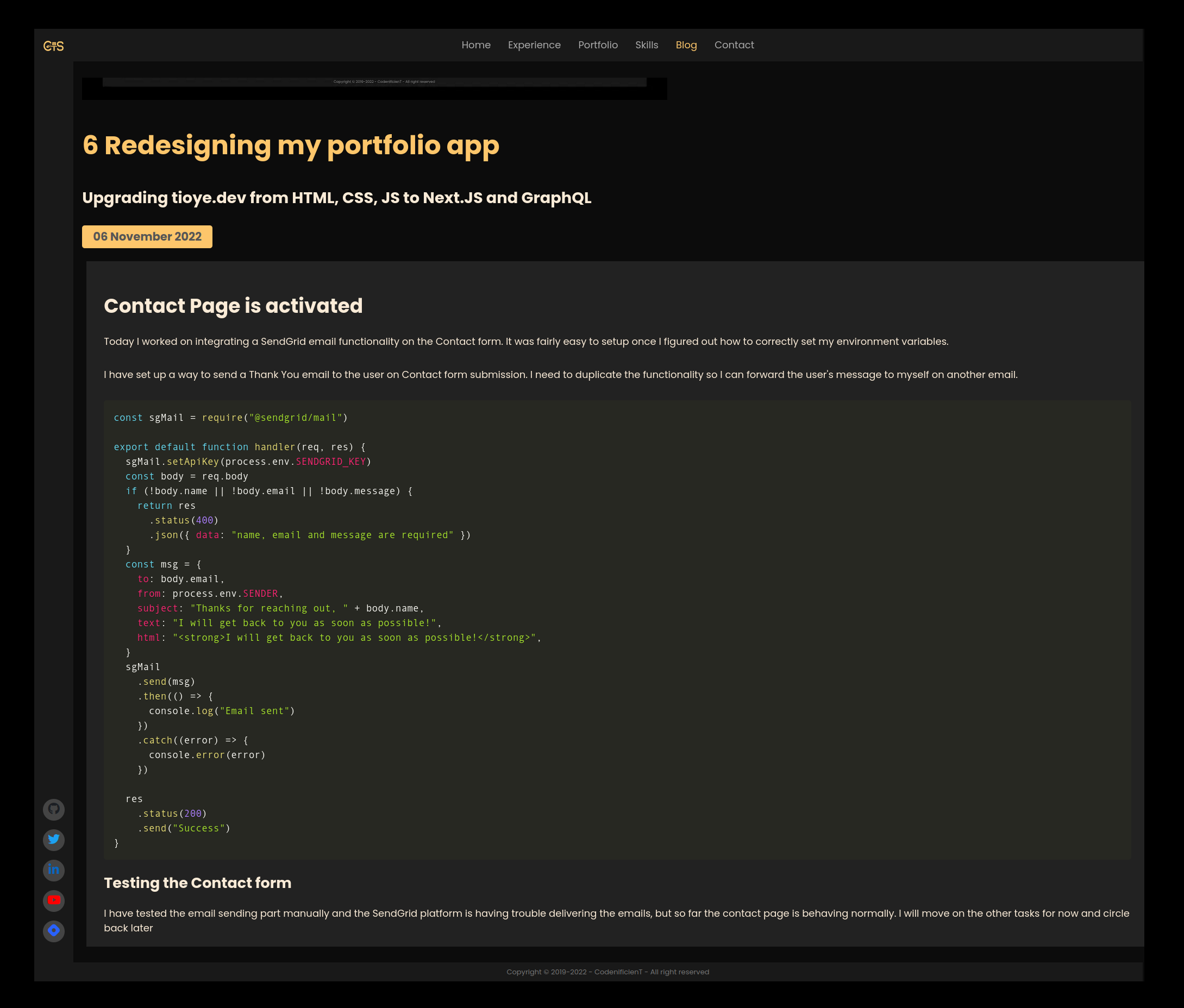